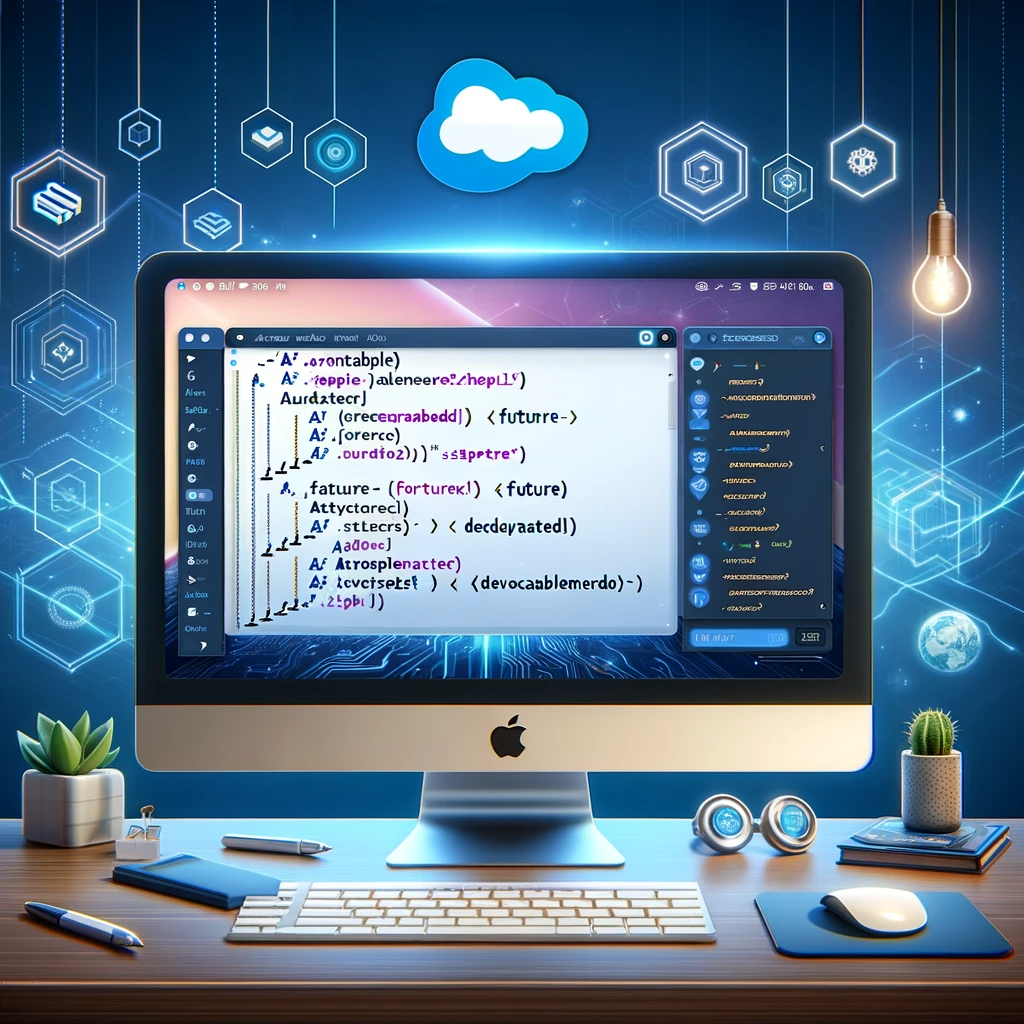
@AuraEnabled
Purpose: Makes a method accessible from a Lightning component.
Example:
public class MyController {
@AuraEnabled
public static String sayHello(String name) {
return 'Hello ' + name;
}
}
@Deprecated
Purpose: Indicates that a method or class should no longer be used.
Example:
public class OldClass {
@Deprecated
public static void oldMethod() {
// Old implementation
}
}
@Future
Purpose: Marks a method that runs asynchronously.
Example:
public class AsyncProcessor {
@Future
public static void processAsync() {
// Asynchronous processing
}
}
@InvocableMethod
Purpose: Allows a method to be called from a Process Builder or Flow.
Example:
apex
Copy code
public class FlowController {
@InvocableMethod
public static void processRecords(List<Id> recordIds) {
// Process logic
}
}
@InvocableVariable
Purpose: Used within a class that has @InvocableMethod to define variables that can be set from a Flow or Process Builder.
Example:
public class Parameters {
@InvocableVariable
public String param1;
}
@IsTest
Purpose: Marks a class or method as a test class or test method.
Example:
@IsTest
private class TestMyClass {
@IsTest static void testMyMethod() {
// Test code
}
}
@JsonAccess
Purpose: Allows non-public members of Apex classes to be serialized and deserialized in JSON.
Example:
public class MyClass {
@JsonAccess
private String myField;
}
@NamespaceAccessible
Purpose: Exposes a global class, method, or variable to any Apex code that resides in a namespace.
Example:
@NamespaceAccessible
public class SharedClass {
// Class implementation
}
@ReadOnly
Purpose: Allows a Visualforce page to perform read-only operations that do not count against the governor limits for SOQL queries.
Example:
@ReadOnly
public with sharing class MyReadOnlyClass {
// Query logic
}
@RemoteAction
Purpose: Exposes a method in a Visualforce controller to be called from JavaScript.
Example:
public with sharing class MyController {
@RemoteAction
public static String performAction(String param) {
// Action logic
}
}
@SuppressWarnings
Purpose: Instructs the compiler to suppress specific warnings.
Example:
@SuppressWarnings('deprecation')
public class MySuppressedClass {
// Code that uses deprecated methods
}
@TestSetup
Purpose: Used in test classes to define methods that set up test data.
Example:
@IsTest
private class MyTestClass {
@TestSetup
static void setup() {
// Setup test data
}
}
@TestVisible
Purpose: Allows test methods to access private or protected members of another class.
Example:
public class MyClass {
@TestVisible private static Integer myCounter = 0;
}
Each of these annotations serves a specific purpose and helps define the behavior of your Apex code in different scenarios, particularly in the context of Salesforce's multi-tenant environment and its governor limits.